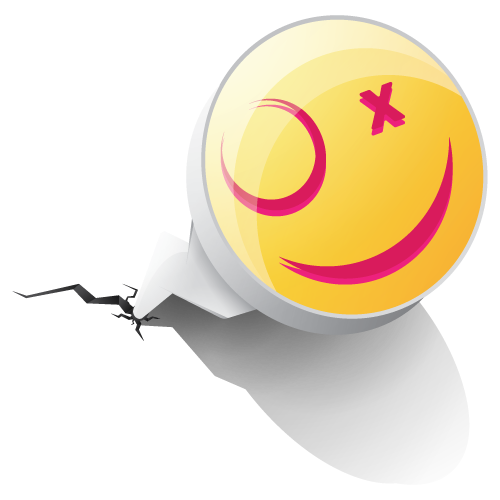
Developer Skills and Editor Setup
Setting up Prettier and VS Code
Without going into the nitty-gritty of what Prettier is, let's keep it short and say Prettier is an opinionated formatter, it helps to format your code to a standard look.
To set up Prettier, we first need to install the extension inside VS Code and then set it as the default formatter within our settings.
{
"arrowParens": "avoid",
"singleQuote": true,
"htmlWhitespaceSensitivity": "ignore",
"bracketSameLine": true
}
We then need to create a '.prettierrc' file that we can set some rules for our preferred formatting style. More style or rules can be found here on Prettier's webpage . Other extensions we can install is eslint and TODO highlights.
VS Code snippets is another great tool to help your workflow. By creating snippets of commonly used commands, such as typing 'cl' for 'console.log( ... )', can help for repetitive tasks.
To navigate and set up our snippets, go to File > Preferences > Configure User Snippets > New Global Snippet. From there follow the example snippet.
Installing Node.js and setting up a dev environment
Installing Node.js and setting up a dev environment.
The first thing we need to do is install node.js, This can be achieved multiple different ways. Using the browser, we can download it directly from the node.js webpage Node.js .
// For windows users
-> winget search node
//Expected output is a list of packages
// Find a package (name)Node.js LTS -- (id)OpenJS.NodeJS.LTS
-> winget install OpenJS.NodeJS.LTS
// Test version
node -v
// Expected output at this time should be v18.12.1
Another method is using the terminal and, depending on your OS, check your package manager for node.js.
-> npm install live-server -g
The last thing is to install live-server globally using npm. npm means Node Package Manager.
Learning how to code
To understand how to code, we first need to learn how we can fail at coding. The first failure when it comes to coding is when you do not have a clear goal for what you want to achieve.
Copying and pasting code, without understanding how or why the code works the way it does, is one of the worst practices you can get into when learning how to code.
No note-taking, or practicing what was done within the course and especially outside the course on your own.
Getting frustrated with not having cleaner code and losing motivation because you feel like you cannot know everything. Learning by yourself and not being able to build anything on your own.
The solution to this is to set a goal for yourself, don't copy and paste code, but try writing it out. Reinforce what you learn by doing small projects on your own and practicing with sites like Codewars to help with challenges.
Coding your own projects is really the best way to reinforce what you learn. While learning how to code, don't bother or worry about clean code, just code and the rest will come over time.
How to think like a developer — Become a problem solver
There are 4 steps to solving any problem and that is to understand the problem, divide and conquer, research and pseudocode. An example to work with is, we are asked to make a function that reverses whatever we pass into it.
Step 1 Understanding the problemUnderstand the problem and ask the right questions to get a clearer picture. While we still in our beginning stages, we won't know what the correct questions will be.
Regarding the example above, What should be reversed? Data types like strings, numbers and arrays make sense to reverse or are reversible compared to something like an object that isn't structured.
What happens when something else is passed into the function?,What should we return, the same value or just a string? How do we recognize if what is passed in is a number or a string? And how do we reverse a number or string or array?
Step 2 Divide and ConquerBreak a big problem into smaller problems! Check if the argument is a number or a string or an array. Implement reversing a number, string or array and return the reversed value.
Step 3 ResearchDon't be afraid to research, if we can't do it by ourselves, which we should always try to do first, then there is no shame in research how to do something.
We can use sites like Google , Stack Overflow , MDN to help us in our research.
Step 4 Using Pseudo Code
function reverse (value)
if value !string and !number and !array
then return the value
Though
if value type === string
then return reversed string
if value type === number
then return reversed number
if value type === array
then return reversed array
return reversed value
Lastly, we can write pseudocode to understand something clearer, especially if the project is large.
Using Google, StackOverflow and MDN
// Problem 1
const temperatures = [3, -2, -6, -1, 'error', 9, 13, 17, 15, 14, 9, 5];
const temperatures2 = [-3, -2, -6, -1, 'error'];
const temperatures3 = ['error', -3, -2, -6, -1]; // This will result in a bug
const tempAmplitude = function (arr) {
let max = arr[0];
let min = arr[0];
for (let i = 0; i < arr.length; i++) {
const curTemp = arr[i];
if (typeof curTemp !== 'number') {
continue;
} else if (curTemp > max) {
max = curTemp;
} else if (curTemp < min) {
min = curTemp;
}
}
let amplitude = max - min;
return `Todays amplitude was ${amplitude}`;
};
We work for a company building smart home appliances, specifically home temperature control units. Your project manager provides you a task of calculating the temperature amplitude within a given day. The project manager tells you that there is a possibility for a sensor read 'error'.
Step 1 Understanding the problemWhat is temperature amplitude? It's the difference between the highest and lowest given temperatures.
How to compute the Max and Min temperatures within an array?What's a sensor error and what to do when it appears?
Step 2 Divide and ConquerFind the max and min values in an array. Ignore the errors when the occur, return the amplitude value
// Problem 2
const tempAmplitude = function (arrOne, arrTwo) {
const arr = arrOne.concat(arrTwo);
let max = arr[0];
let min = arr[0];
for (let i = 0; i < arr.length; i++) {
const curTemp = arr[i];
if (typeof curTemp !== 'number') {
continue;
} else if (curTemp > max) {
max = curTemp;
} else if (curTemp < min) {
min = curTemp;
}
}
console.log(max, min);
let amplitude = max - min;
return `Todays amplitude was ${amplitude}`;
};
console.log(tempAmplitude(temperatures, temperatures2));
You finish what was required of you, and you report your work to your project manager, but there is a problem, your manager was told that they have upgraded their prototype, and the device now outputs 2 arrays. You are now tasked with making that same function work with 2 arrays instead of 1.
Step 1 Understanding the problemShould we just implement the function twice? No, just merge the arrays into a single array.
Step 2 Divide and ConquerMerge 2 arrays into 1.
Debugging — Fixing errors
A software bug is any unintended or unexpected behavior of a software application. The term 'bug' was coined by Harvard when one of their computers actually had a bug inside it.
How does one detect bugs? This usually happens during the development stages, during testing and from user reports during production and in some case's just by context, like certain browsers and only specific users.
Once we have identified the bug, we need to find it and then fix it, as well as prevent it from happening again. With smaller code, using the console can be an easy way of debugging apps. However, if the code is larger, we use a debugger. Once fixed, we try to prevent it from happening again.
When it comes to debugging, your mantra should be to Identify, Find, Fix and Prevent.
Debugging with the console and breakpoints
// Example Code Here
console.log()
console.warn()
console.error()
console.table() // which is great for displaying objects
Before we get into practicing debugging, there are some nice console.log alternatives, which are really just for aesthetic purposes. The console.table(object) is really helpful when it comes to displaying objects.
// This code outputs a result of 10273
// Expected result should be 283
const measureKelvin = function () {
const measurement = {
type: 'temp',
unit: 'Celsius',
// value: prompt('Degree Celsius')
value: '10',
};
const kelvin = measurement.value + 273;
return kelvin;
};
console.log(measureKelvin());
You can also use the browser's debugger by going to sources and setting a breakpoint for where you want your code to stop. This way of debugging is really handy for loops.
// This code outputs a result of 283
const measureKelvin2 = function () {
const measurement = {
type: 'temp',
unit: 'Celsius',
// value: parseInt(prompt('Degree Celsius'))
value: 10,
};
const kelvin = measurement.value + 273;
return kelvin;
};
console.log(measureKelvin2());
Coding challenge - 1
Given an array of forecasted maximum temperatures, the thermometer displays a string with the given temperatures. Example: [17, 21, 23] will print "... 17ºC in 1 days ... 21ºC in 2 days ... 23ºC in 3 days ..."
Your Tasks:-
Create a function 'printForecast' which takes in an array 'arr' and logs a string like the above to the console. Try it with both test datasets.
-
Use the problem-solving framework: Understand the problem and break it up into sub-problems!
-
Data 1: [17, 21, 23]
-
Data 2: [12, 5, -5, 0, 4]
const maxTemps1 = [17, 21, 23];
const maxTemps2 = [12, 5, -5, 0, 4];
const printForecast = function (arr) {
let logTemp = '';
for (let i = 0; i < arr.length; i++) {
const curTemp = arr[i];
logTemp += `... ${curTemp}°C in ${i + 1} days `;
}
return logTemp;
};
console.log(`${printForecast(maxTemps1)}...`);
console.log(`${printForecast(maxTemps2)}...`);
From a single array, print a string to the console, is the string single or multiple lines? The answer is that it's a single line with three dots as spaces.The information or input is from an array. A single string needs to store x number of values, that are different.
Divide and ConquerCreate a function that's in an array of values (in this case, the maximum temperatures). Loop over the array, and each iteration should add a string to an empty variable. The string should be as long as the input.