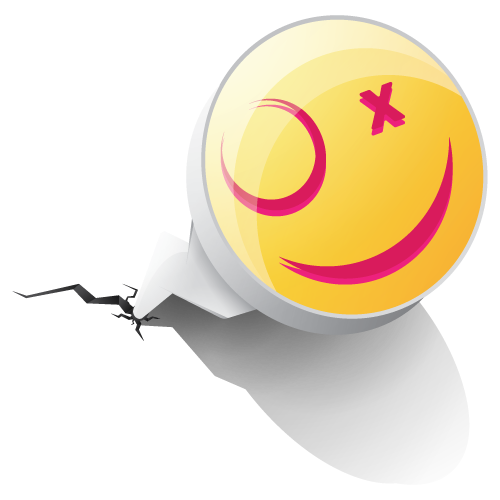
A closer look at functions
Default Parameters
// Default parameters
const bookings = [];
const createBooking = function (
flightNum,
numPassenger = 1,
price = 199 * numPassenger
) {
// Old way of setting default parameters
// numPassenger = numPassenger || 1;
// price = price || 199;
const booking = {
flightNum,
numPassenger,
price,
};
console.log(booking);
bookings.push(booking);
};
createBooking('LH123');
createBooking('LH123', 1, 499);
createBooking('LH123', 2);
createBooking('LH123', 5);
createBooking('LH123', undefined, 399);
Above is how we can set default values for parameters in functions as well as make dynamic default parameters, though it's important to remember that JavaScript read these parameters from left to right.
We also take a look at how to skip parameters by using the keyword 'undefined'. We can think of this much like the array deconstruction, though instead of placing an empty space, we simply use the word undefined.
How Passing Arguments Works: Value vs. Reference
const flight = 'LH999';
const andre = {
name: 'Andre Coetzer',
passport: 1122334455,
}
const checkIn = function (flightNum, passenger) {
flightNum = 'LH555';
passenger.name = 'Mr. ' + passenger.name;
if (passenger.passport === 1122334455) {
alert('Checked In');
} else {
alert('Wrong Passport')
}
}
checkIn(flight, andre)
console.log(flight);
console.log(andre.name);
Above, we demonstrate how function can affect values and reference types. Like we discussed before, primitive values won't change but reference type will, as how it behaves within the memory heap.
When passing a value or object to a function it's really as if we are copying the object like so, "const flightNum = flight".
// example of how things can go wrong
const newPassport = function (person) {
person.passport = Math.trunc(Math.random() * 1000000000);
}
newPassport(andre)
checkIn(flight, andre)
console.log(andre.passport);
Above, we show how something like this can cause issues on bigger code bases with multiple developers.
First-Class and Higher-Order Functions
// Example Code Here
CHANGE ME
Functions Accepting Callback Functions
// Example Code Here
CHANGE ME
Functions Returning Functions
// Example Code Here
CHANGE ME
The call and apply Methods
// Example Code Here
CHANGE ME
The bind Method
// Example Code Here
CHANGE ME
Coding Challenge #1
// Example Code Here
CHANGE ME
Immediately Invoked Function Expressions (IIFE)
// Example Code Here
CHANGE ME
Closures
// Example Code Here
CHANGE ME
More Closure Examples
// Example Code Here
CHANGE ME
Coding Challenge #2
// Example Code Here
CHANGE ME