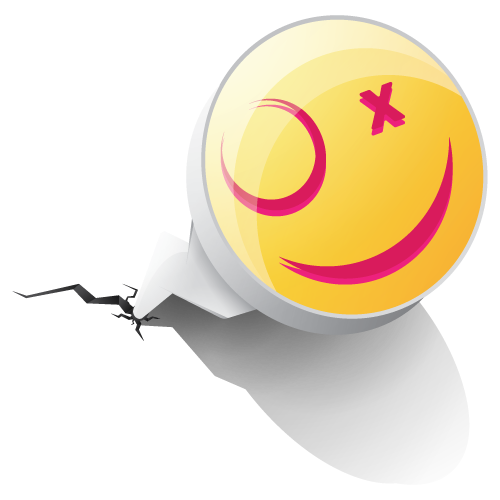
JavaScript fundamentals (Part 1)
Hello, World!
Welcome to JavaScript, before we write anything in our editor, we will take a look at some basic JavaScript by using the browser's console.
To open the console within your browser, there are a few ways you could achieve this.
-
Right Click
Right click somewhere on the browsers window and select inspect and navigate to the tab that says console.
-
Shortcut
The second method is to press Ctrl Shift + J, which should bring up the console directly.
-
Settings
Lastly, depending on your browser, somewhere in its menu should be the developer tools.
Inside the console, we can take a look at some basic commands, which will be explained at a later time. The first thing we will learn is all famous βHello, World!β which is a rite of passage for anyone learning code.
alert('Hello, World!')
If we write or copy this code above into our browser's console, we should see a popup appear at the top of the screen. Let's take a look at some other things JavaScript can do with alert while we are in the browser.
// Defining a Variable
let js = 'amazing'
// Creating basic logic
if (js === 'amazing') alert('JavaScript is Fun!')
Above, we first defined a variable using let, more on this later, and then used an if statement with an equality operator to create some form of logic. In pseudocode, it's if the variable's value is strictly equal to the string amazing, then do this. The 'this', is the alert function that will be run.
If we copy and paste the code above in your console, you should see the popup with the words 'JavaScript is fun!'. Lets to change the variable to something else, and see what happens.
// Defining a Variable
let js = 'boring'
// Creating basic logic
if (js === 'amazing') alert('JavaScript is Fun!')
Now, if we copy the copy above, you should see nothing happening. That is because the if statement returns false instead of true, and therefor does not run the code.
A brief introduction to JavaScript
JavaScript is considered to be a high level, object-oriented, multi paradigm, programming language. Starting with programming language, JavaScript is the programming language for the web. The meaning of high level is that, we don't have to worry about things like ram usage among other things, we can simply focus purely on programming.
It's object orientated because most things are done with and inside of objects. It's also multi paradigm in the sense that many programming styles can be used in JavaScript.
If we look at what makes a website, we can place together HTML, CSS, and JavaScript. HTML, provides the content on the page, otherwise known as the Noun, CSS is the appearance, also known as the Adjective, while JavaScript is the programming language for the site, also known as the Verb.
<!-- The Noun -- >
<p>
Paragraph
</p>
/* The Adjective */
p {
font-size: 16px;
}
// The Verb
p.hide();
JavaScript allows for both frontend programming and backend using technologies such as Node.js. JavaScript has its libraries such as React, Angular and Vue which are ever-changing and also sometimes easier to use than plain JavaScript.
That's not all, there is also React Native and Ionic that can be used for Desktop applications as well as mobile. The possibilities are almost endless.
Linking a JavaScript file
Linking a .js file works similar to that of linking a CSS file. The only difference is that it is best practice to add the JavaScript file just before the end of the body HTML element, whereas the .css file is linked within the head HTML element. You could however write an internal script, just like an internal style for CSS, however it's not preferred for cleaner code.
An internal script would look something like the following, below.
<!DOCTYPE html>
<html lang="en">
<head>
<!-- The Internal Method -- >
<script>
// JavaScript In here
console.log('Hello');
</script>
</head>
<body>
</body>
</html>
While if external, it would require you to make an app.js file and then you to link it to your HTML document like the following below.
<!DOCTYPE html>
<html lang="en">
<head>
</head>
<body>
<!-- The External Method -- >
<script src="./resource/javascript/app.js"></script>
</body>
</html>
Now that we will not be working in the console, to log values to the console directly, we can still use the console to output some values using a command in JavaScript called console.log() as shown below.
// To log something to the console
console.log('π This is how to log to the console!');
If you view the console, you should see a similar message displayed. Of course, we did this using an external .js file which we linked to this page.
Values and Variables
'Andre' // This is a value
console.log('Andre');
Above is what is known as a value in JavaScript. A value is the smallest unit of information inside of JavaScript. Though, to can see this value, we would need to log this to the console.
let firstName = 'Andre';
Above is called declaring a variable, where 'firstName' is the variable's name, it's then assigned a value and holds information inside it. A variable is much like a box in the real world, where you can place a book inside the box and label the box 'book', for example. This works much the same way.
The variable will take whatever value or data you place inside it and store it in your computer's memory so that if you want to use it at a later date, you can. To use a variable, all you need to do is call on that variable's name, Instead of writing out the literal value.
console.log(firstName);
When it comes to naming variables, there are a few naming conventions as well as hard rules to follow. So far we have been using what is known as camel casing, where the first word and letter is lowercase and all subsequent words are capitalized thereafter. Another method is called snake casing and is very prominent with in the Ruby community. Snake casing separates each word with an underscore.
// Camel Casing
let firstName;
// Snake Casing
let first_name;
Variables names cannot start with a number, this is seen as something illegal within JavaScript. Though it can contain a number, just not as the first letter. In fact, a variable can only contain the following, numbers, letters, underscores, and the dollar symbol.
Respectfully, variable names cannot be the same as reserved keywords within JavaScript. An example of that would be the keyword new. There is one keyword that is not illegal but by convention is not used by itself when naming variables, and that is the keyword name.
Furthermore, Variables as per convention should not start with a capital letter, as these variables are use for something called object-oriented programming. Adding to this, variables should also not be in all uppercase letters, as these are reserved for constants that we know will never change.
Lastly, variables should be descriptive, for example below.
// Non descriptive naming
let job1 = 'Graphic Designer'
let job2 = 'Web Developer'
// More descriptive naming
let firstJob = 'Graphic Designer'
let secondJob = 'Web Developer'
Practice Assignment
-
Declare variables called 'country', 'continent' and 'population' and assign their values according to your own country (population in millions)
-
Log their values to the console
const country = 'Bulgaria';
const continent = 'Europe';
let population = 6899000; // Don't put spaces
console.log(`${country}, ${continent}, ${population}`)
Practice assignments
As seen above, each section has an assignment, you can view the results inside the console. Note that after each section, check the assignment .pdf to see what assignment is given.
Data Types
// An object
let me = {
name: 'Andre';
age: 33;
}
// Primitive
let firstName = 'Andre';
let age = 33;
In JavaScript, every value is either an object or a primitive. There are 7 primitive data types. Number, Strings, Boolean, Undefined, Null, Symbol and Big Int.
// Number Data Type
let age = 33; // is actually 33.0
The number data type, is always a floating point number, even though we don't see a decimal, it is there. Unlike other programming languages, we don't need to specify whether a number is a float or int.
// String Data Type
let firstName = 'Andre'; // Single quotation marks
let firstName = "Andre"; // Double quotation marks
let firstName = `Andre`; // String template literals
let firstName = "Andre'; // Mixing not allowed!
Strings take a sequence of characters within its quotation marks. The quotation marks can be either double or single, but you cannot mix them.
// Boolean Data Type
let isStudying = true;
let isSleeping = false;
Booleans consist of only 2 values, true or false. This data type is used for decision making in our code.
// Undefined Data Type
let lastName;
console.log(lastName)
console.log(typeof lastName)
An undefined data type is when a variable takes the type undefined when it has an empty value. Just to add, undefined is both a value and a data type. We can see this by the code above.
Small note on typeof, as we will see later, it is an operator and is used to see the type a value is.
Null is very similar to undefined, though not the same. More on this later in the course.
Symbol, is a value that will never change, again more on this later.
Big Int, the same as number but allows for larger numbers. More on this later in the course.
// JavaScript as a dynamic language
let javascript = true;
console.log(javascript);
javascript = 'YES!'
console.log(javascript)
JavaScript is a dynamic language, as seen above we do not need to declare the variable twice, though just reassign the value.
Practice Assignment
-
Declare a variable called 'isIsland' and set its value according to your country. The variable should hold a Boolean value. Also declare a variable 'language', but don't assign it any value yet
-
Log the types of 'isIsland', 'population', 'country' and 'language' to the console
// Working with the last assignment in mind
const isIsland = false;
let language;
console.log(typeof country, typeof population, typeof isIsland, typeof language);
let, const and var
let age = 33;
const birthYear = 1989;
var outOfDate = true; // var is legacy
In JavaScript, there are 3 ways of declaring variables. That being let, const and the legacy way, var. The difference between let and const is that let is mutable and const is not. This means that let can change its value or even data type to something completely different, and it's legal in JavaScript.
Const however cannot do this and is not mutable. Therefore, you cannot reassign or mutate the value or data type inside a const variable. It's basically constant. You also shouldn't leave a const variable undefined.
How do we know which to use? When it comes to best practice, it's best to only use const, unless you know the value will change later in your code. It's also a best practice to not introduce too many mutable variables into your code, as this can lead too hard to find bugs.
Var on the other hand should be avoided and no longer used, as it's the legacy way of declaring a variable. It's only here to know how it works, just in case you encounter one in the wild.
Practice Assignment
-
Set the value of 'language' to the language spoken where you live (some countries have multiple languages, but just choose one)
-
Think about which variables should be const variables (which values will never change, and which might change?). Then, change these variables to const.
-
Try to change one of the changed variables now, and observe what happens.
// Reassigning the value of language
language = 'Bulgarian'
isIsland = true; // Assigned to constant ERR
Basic operators
There are many operators in JavaScript. Arithmetic, Comparison, Logical, Assignment and many more. Operators allow us to transform multiple different values, or combine them.
// Arithmetic operators
console.log(2 + 1) // Addition
console.log(3 - 2) // Subtraction
console.log(4 * 2) // Multiplication
console.log(6 / 2) // Division
console.log(2 ** 2) // Exponents
console.log(3 % 2) // Modulo (Remainder)
const now = 2022;
const ageAndre = now - 1989;
const ageSammy = now - 1991;
console.log(ageAndre, ageSammy)
// String concatenation
const myText1 = 'I love'
const myText2 = 'JavaScript'
console.log(myText1 + ' ' + myText2)
Arithmetic operators operate as you would expect them too. You can use the plus + operator to concatenate strings together. In fact, you can concatenate different data types like together in a process known as type coercion, which we will cover later. There is a better way of concatenating strings together, called String template literals.
let x = 10 + 20;
x += 10; // which means x = x + 10
x -= 10; // which means x = x - 5
// Incremental
x++; // which means x = x + 1
// Decremental
x--; // which means x = x - 1
Assignment Operators, as you would have guessed it, assign something like a value to a variable using the equal's syntax. Above, we see a variable x being declared and assigned a value in the form of a mathematical equation.
As we will understand later on, that mathematical equation is what is known as an expression. An expression in JavaScript is anything that returns a value, more on this later. What's important to know here is that there is something called operator precedence, which again we will learn more about later.
Though in short, the equation will execute first, providing a value. That value would in return be assigned to the x variable.
Following that, are a few more assignment operators, most importantly the Incremental and Decremental, as this we use quite often.
// Comparison operators
age1 > age2 // Greater than
age1 < age2 // Less than
age1 >= age2 // Greater than or Equal to
age1 <= age2 // Greater than or Equal to
let age1 = 18;
let age2 = 33;
let age3 = 33;
console.log(age1 > age2);
console.log(age1 < age2);
console.log(age2 >= age3);
console.log(age2 <= age3);
Comparison operators return a boolean value, they are used in the decision-making process.
Practice Assignment
-
If your country split in half, and each half would contain half the population, then how many people would live in each half?
-
Increase the population of your country by 1 and log the result to the console
-
Finland has a population of 6 million. Does your country have more people than Finland?
-
The average population of a country is 33 million people. Does your country have less people than the average country?
-
Based on the variables you created, create a new variable 'description' which contains a string with this format: 'Portugal is in Europe, and its 11 million people speak portuguese'
let halfOfPopulation = population / 2;
console.log(halfPopulation)
console.log(population);
population++
console.log(population)
let finland = 6000000;
console.log(population > finland);
let averagePopulation = 33000000;
console.log(population < averagePopulation);
// String concatenation
let description = country + ' is in ' + continent + ", and it's " + population + ' people speak ' + language;
// String template literals
let description2 = `${country} is in ${continent}, and it's ${population} people speak ${language}`;
Operator precedence
Different operators in JavaScript have higher precedence over others. We can see that here in the precedence table provided by MDN. On the table, we can see the mathematical operators have a higher precedence over the assignment operator. This explains why x had a value of 30, and that is because the mathematical operator had precedence over the assignment operator.
let x = y = 25 - 10 - 5; // Both equal 10 10
Certain operators also work from right to left, as in the example above, both x and y will equate to 10. If we break it down we understand that the equation happens first, then the value of that expression is assigned to the variable y which is then assigned to the variable x because it works from right to left. If this was reversed, the entire statement and expression would not work.
Coding challenge — 1
Mark and John are trying to compare their BMI (Body Mass Index), which is calculated using the formula: BMI = mass /height ** 2 = mass / (height * height) (mass in kg and height in meter)
Your Tasks:-
Store Mark's and John's mass and height in variables
-
Calculate both their BMIs using the formula (you can even implement both versions)
-
Create a Boolean variable 'markHigherBMI' containing information about whether Mark has a higher BMI than John.
-
Data 1: Marks weights 78 kg and is 1.69 m tall. John weights 92 kg and is 1.95m tall.
-
Data 2: Marks weights 95 kg and is 1.88 m tall. John weights 85 kg and is 1.76m tall.
// Data 1
/*
const weightMark = 78;
const heightMark = 1.69;
const weightJohn = 92;
const heightJohn = 1.95;
*/
// Data 2
const weightMark = 95;
const heightMark = 1.88;
const weightJohn = 85;
const heightJohn = 1.76;
const bmiMark = weightMark / heightMark ** 2;
const bmiJohn = weightJohn / (heightJohn * heightJohn);
const markHigherBMI = bmiMark > bmiJohn ? "Mark's is higher" : "John's is higher";
console.log(`Mark's BMI is ${bmiMark}, John's BMI is ${bmiJohn} and is Mark's BMI higher than John's? : ${markHigherBMI}`);
Strings and template literals
const myName = 'Andre';
// String concatenation
console.log('Hello, my name is ' + myName);
// String template literals
console.log(`Hello, my name is ${myName}`)
String template literals allows you to not do string concatenation anymore. You can now inject the variable directly into your string. You can imagine this is very useful in creating cleaner and more understanding code.
A template literal or string template literal is different to the quotation marks as it uses the back tick character. A lot of developers prefer this over the quotation marks as they don't need to worry about which to use. Though, there could be performance issues if you just use template literals.
Practice Assignment
-
Recreate the 'description' variable from the last assignment, this time using the template literal syntax.
// String template literals
let description3 = `${country} is in ${continent}, and it's ${population} people speak ${language}`;
Taking decisions — if and else statements
// If statement
if (condition) {
console.log(something)
} else if (condition) {
console.log(something else)
} else {
console.log(nothing)
}
// Whats known as a control structure
if (condition) {
console.log(something)
} else {
console.log(nothing)
}
Above is an example of an if statement, an if statement is used to make decisions. Let's start by making a decision with JavaScript by making a program that lets us know whether a person is old enough to get their driver's license and if not, how many years until they are allowed.
let age = 20;
if (age >= 18) {
console.log('Is allowed to drive π')
} else {
const yearsLeft = age - 18;
console.log(`Sorry, you have to wait ${yearsLeft} years before to can get your drivers license`)
}
Practice Assignment
-
If your country's population is greater that 33 million, log a string like this to the console: 'Portugal's population is above average'. Otherwise, log a string like 'Portugal's population is 22 million below average' (the 22 is the average of 33 minus the country's population)
-
After checking the result, change the population temporarily to 13 and then to 130. See the different results, and set the population back to original
if (population > averagePopulation) {
console.log(`${country} population is above average.`)
} else {
console.log(`${country} population is ${averagePopulation - population} below the average population`)
}
Code challenge — 2
Use the BMI example from Challenge #1, and the code you already wrote, and improve it.
Your Tasks:-
Print a nice output to the console, saying who has the higher BMI. The message is either "Mark's BMI is higher than John's!" or "John's BMI is higher than Mark's!"
-
Use a template literal to include the BMI values in the outputs. Example: "Mark's BMI (28.3) is higher than John's (23.9)!"
if (bmiMark > bmiJohn) {
console.log(`Mark's BMI(${bmiMark}) is higher than John's BMI(${bmiJohn})`)
} else {
console.log(`John's BMI(${bmiJohn} is higher than Mark's BMI(${bmiMark}))`)
}
Type conversion and coercion
In programming, converting between data types is something that happens often. Whether it is from a string to number or vice versa. In JavaScript, we call this type conversion and type coercion, the difference between the 2 being the first is explicit while the second is implicit.
Type conversion is a manual conversion of a type, while Type coercion is JavaScript automatically converting the type.
There are really only 3 types that can be converted to, that being Number, String, and Boolean. When it comes to Boolean, this is what is known as Truthy and Falsy Values, which will see later on.
// Type conversion using Functions
const inputYear = '1991';
console.log(Number(inputYear));
console.log(String(23), 23);
console.log(Number('Andre')); // will result in NaN
console.log(typeof NaN)
Above is an example of Type conversion where we manually convert the types using functions. One thing to take note of is the result of NaN, if we look at the typeof NaN, it results in a number, even though it stands for Not a Number.
// Type coercion
// To String
console.log('5' + 3 + '2') // Will result in 532
// To Number
console.log('5' - 3 - '2') // Will result in 0
console.log(5 * '2') // Will result in 10
console.log(5 / '2') // Will result in 2.5
// Guess the answer
let n = '1' + 1;
n -= 1;
n = ?
2 + 3 + 4 + '5' = ?
'10' - '4' - '2' - 2 + '5' = ?
With Type coercion, JavaScript automatically converts the type based on some operators. When the expression contains a + JavaScript will convert the type to a string, if it's a - it will convert the expression to a number, the same for * and / as those are the only way those operators can work.
When understood correctly, coercion will allow us to use a lot less code, all we need to do is trust that JavaScript will convert the type correctly.
Practice Assignment
-
Predict the result of these 5 operations without executing them:
'9' - '5';
'19' - '13' + '17';
'19' - '13' + 17;
'123' < 57;
5 + 6 + '4' + 9 - 4 - 2;
-
Execute the operations to check if you were right
console.log('9' - '5'); // 4
console.log('19' - '13' + '17'); // 617
console.log('19' - '13' + 17); // 23
console.log('123' < 57); // false
console.log(5 + 6 + '4' + 9 - 4 - 2); // 1143
Truthy and falsy values
// Falsy values
console.log( 0, '', undefined, null, NaN, false);
console.log(Boolean(0));
console.log(Boolean(' '));
console.log(Boolean(undefined));
console.log(Boolean(null));
console.log(Boolean(NaN));
In JavaScript there are only 5 so called falsy values, the 6th being false of course, everything else is truthy. The understanding of falsy values is that it's not false unless converted to a boolean.
// Falsy values
console.log(Boolean(''));
// Truthy values
console.log(Boolean('Am i falsy?'));
A truthy value is any value that is not falsy, an example of this is a string that is not empty. We can and have seen whether something is truthy or falsy by using the boolean function. However, we never really use this function as these truthy or falsy values are implicit and happens with coercion.
The question is, when does JavaScript do type coercion towards Boolean, and btw we spoke before about type conversion having 3 types it converts to, String, Number, and Boolean. The function above Boolean() is the manual type conversion to Boolean.
let hasMoney = 0;
if (hasMoney) {
console.log('Don't spend it all!');
} else {
console.log('Get a job!') // This will be the outcome
}
To answer the question, JavaScript does coercion in 2 scenarios, when using a logic operator, or in a logical context like the statement above. The above statement will execute the else block, but why? We haven't specified what is and what is not true, though through Truthy and Falsy JavaScript already knows what is true and what is false.
Equality Operators == vs. ====
// Equality operators
=== // Strict equality operator
== // Loose equality operator
// Different operators
!== // Strict not equality operator
!= // Loose not equality operator
Equality operators are part of the comparison operators and provide a boolean value. The only difference between each equality operator is Strict vs Loose.
// Strict
console.log('1' === 1)
console.log(1 === 1)
// Loose
console.log('1' == 1);
console.log(1 == 1);
Looking at the example above we see that with strict, no coercion takes places, whereas with loose JavaScript does a type coercion and matches something called the Unicode values together.
For best practice, we should also try to use the strict equality, as when using loose can result in unwanted bugs.
Practice Assignment
-
Declare a variable 'numNeighbors' based on a prompt input like this: prompt('How many neighbor countries does your country have?');
-
If there is only 1 neighbor, log to the console 'Only 1 border!' (use loose equality == for now)
-
Use an else-if block to log 'More than 1 border' in case 'numNeighbors' is greater than 1
-
Use an else block to log 'No borders' (this block will be executed when 'numNeighbors' is 0 or any other value)
-
Test the code with different values of 'numNeighbors', including 1 and 0.
-
Change == to ===, and test the code again, with the same values of 'numNeighbors'. Notice what happens when there is exactly 1 border! Why is this happening?
-
Finally, convert 'numNeighbors' to a number, and watch what happens now when you input 1
-
Reflect on why we should use the === operator and type conversion in this situation
const numNeighbors = prompt('How many neighbor countries does your country have?')
if (Number(numNeighbors) === 1) {
console.log('Only 1 border');
} else if (numNeighbors > 1) {
console.log('More than 1 border');
} else {
console.log('No border');
}
Boolean Logic
Boolean logic consists of the And, Or and Not Logic operators. It uses various logical operators to combine true or false values. The most important to note about each is the And needs both values to be true in order for the statement to be true. Quick note, here I only mention 2, but there can be more than 2 statements and in this case, all statements need to be true to result in true.
The Or boolean logic needs only 1 statement to be true in order to be true, but both statements to be false in order to be false.
The Not boolean logic, just reverses the value. If it was true, Not would reverse or change that to false.
Logical operators
// Logical operators
something && something // The AND Logical Operator
something || something // The OR Logical Operator
!something // The NOT Logical Operator
This is how the syntax looks, the theory behind it falls under boolean logic. Though, we can build a small program that checks to see if a person is able to drive, and if not lets them know someone else should drive.
// Logical operators
const hasLicense = true;
const hasGoodVision = true;
const isDrunk = false;
if (hasLicense && hasGoodVision && !isDrunk) {
console.log('Is able to drive, YAY!');
} else {
console.log('Someone else should drive..');
};
Practice Assignment
-
Comment out the previous code so the prompt doesn't get in the way.
-
Let's say Sarah is looking for a new country to live in. She wants to live in a country that speaks english, has less than 50 million people and is not an island.
-
Write an if statement to help Sarah figure out if your country is right for her. You will need to write a condition that accounts for all of Sarah's criteria. Take your time with this, and check part of the solution if necessary.
-
If yours is the right country, log a string like this: 'You should live in Portugal :)'. If not, log 'Portugal does not meet your criteria :('
-
Probably your country does not meet all the criteria. So go back and temporarily change some variables in order to make the condition true (unless you live in Canada :D)
const maxPopulation = 50000000;
if (language === 'english' && population < maxPopulation && !isIsland) {
console.log(`You should live in ${country}`);
} else {
console.log(`Sorry, ${country} does not meet your criteria!`);
};
Coding challenge — 3
There are two gymnastics teams, Dolphins and Koalas. They compete against each other 3 times. The winner with the highest average score wins a trophy!
Your Tasks:-
Calculate the average score for each team, using the test data below.
-
Compare the team's average scores to determine the winner of the competition, and print it to the console. Don't forget that there can be a draw, so test for that as well (draw means they have the same average score)
-
Bonus 1: Include a requirement for a minimum score of 100. With this rule, a team only wins if it has a higher score than the other team, and the same time a score of at least 100 points. Hint: Use a logical operator to test for minimum score, as well as multiple else-if blocks π
-
Bonus 2: Minimum score also applies to a draw! So a draw only happens when both teams have the same score and both have a score greater or equal 100 points.Otherwise, no team wins the trophy
-
Data 1: Dolphins score 96, 108 and 89. Koalas score 88, 91 and 110
-
Data Bonus 1: Dolphins score 97, 112 and 101. Koalas score 109, 95 and 123
-
Data Bonus 2: Dolphins score 97, 112 and 101. Koalas score 109, 95 and 106
// const dolphinsAverage = (96 + 108 + 89) / 3;
// const koalasAverage = (88 + 91 + 110) / 3;
// const dolphinsAverage = (97 + 112 + 101) / 3;
// const koalasAverage = (109 + 95 + 123) / 3;
const dolphinsAverage = (97 + 112 + 101) / 3;
const koalasAverage = (109 + 95 + 106) / 3;
console.log(dolphinsAverage, koalasAverage)
if (dolphinsAverage > koalasAverage && dolphinsAverage >= 100) {
console.log('Dolphins have won')
} else if (koalasAverage > dolphinsAverage && koalasAverage >= 100) {
console.log("Koalas have won")
} else if (dolphinsAverage === koalasAverage && dolphinsAverage >= 100 && koalasAverage >= 100) {
console.log("It's a draw")
} else {
console.log('None have won')
};
The switch statement
const day = 'tuesday';
switch (day) {
case 'monday':
console.log('mow the lawn')
break;
case 'tuesday' :
console.log('clean the pool')
break;
case 'wednesday':
case 'thursday':
console.log('singing lessons')
break;
case 'friday':
console.log('pizza night')
break;
case 'saturday':
case 'sunday':
console.log('relax')
break;
default:
console.log("Ok, that's not in the list")
};
A switch statement is an alternative to writing an if statement, just with less repeated code. It's useful if you wish to compare one value to multiple different options.
Taking a look at the syntax, most is self-explanatory. Some key elements to note is that you can combine cases together, and the default keyword is like the else statement.
const day = 'tuesday';
if (day === 'monday') {
console.log('mow the lawn');
} else if (day === 'tuesday') {
console.log('clean the pool')
} else if (day === 'wednesday' || day === 'thursday') {
console.log('singing lessons')
} else if (day === 'friday') {
console.log('pizza night')
} else if (day === 'saturday' || day === 'sunday') {
console.log('relax')
} else {
console.log("Ok, that's not in the list")
};
The same as the switch statement just in the form of an if statement, we can clearly see the repeated code. However, that being said, the switch statement is not really used anymore and people prefer to use the if statement.
Practice Assignment
-
Use a switch statement to log the following string for the given 'language':
chinese or mandarin: 'MOST number of native speakers!'
spanish: '2nd place in number of native speakers'
english: '3rd place'
hindi: 'Number 4'
arabic: '5th most spoken language'
for all other simply log 'Great language too :D'
switch (language) {
case 'chinese':
case 'mandarin':
console.log('MOST number of native speakers');
break;
case 'spanish':
console.log('2nd place in number of native speakers');
break;
case 'english':
console.log('3rd place')
break;
case 'arabic':
console.log('5th most spoken language');
break;
default:
console.log('Great language too');
}
Statements and expressions
3 + 4 // Is known as an expression
'1991' // Also an expression
When talking about statements and expressions, an expression is essentially something that returns a value, whether it be a math equation like 1 + 1 or a number, string. It's a piece of code that produces a value.
A statement is a larger piece of code that does not produce a value, but a sequence of actions, such as an if statement.
// String literal
console.log(`A string template literal ${expression}`)
The reason it's important to understand the difference is because JavaScript requires us to know where to place them. A good example of this would be the string template literals. It expects something that can produce a value, however you can't place a statement within in.
The conditional ternary operator
// Ternary operator
const someAge = 17;
someAge >= 18 ? console.log('Drink Wine') : console.log('Drink juice box');
The Ternary operator is an expression and not a statement. It only accepts one line of code and returns a value. This means that it can be used within a string template literal.
Practice Assignment
-
If your country's population is greater than 33 million, use the ternary operator to log a string like this to the console: 'Portugal's population is above average'. Otherwise, simply log 'Portugal's population is below average'. Notice how only one word changes between these two sentences!
-
After checking the result, change the population temporarily to 13 and then to 130. See the different results, and set the population back to original
population >= averagePopulation ? console.log(`${country} is above the average population`) : console.log(`${country} is below the average population`);
Coding challenge — 4
Steven wants to build a very simple tip calculator for whenever he goes eating in a restaurant. In his country, it's usual to tip 15% if the bill value is between 50 and 300. If the value is different, the tip is 20%.
Your Tasks:-
Calculate the tip, depending on the bill value. Create a variable called 'tip' for this. It's not allowed to use an if/else statement π (If it's easier for you, you can start with an if/else statement, and then try to convert it to a ternary operator!)
-
Print a string to the console containing the bill value, the tip, and the final value (bill + tip). Example: βThe bill was 275, the tip was 41.25, and the total value 316.25β
-
Data 1: Test for bill values 275, 40 and 430
-
To calculate 20% of a value, simply multiply it by 20/100 = 0.2
-
Value X is between 50 and 300, if it's >= 50 && <= 300 π
const bill = 430;
const tip = bill >= 50 && bill <= 300 ? (bill * 15) / 100 : (bill * 20) / 100;
console.log(tip)
console.log(`The bill amount was ${bill}, the tip will amount to ${tip} and the total is ${bill + tip}`);
JavaScript Releases: ES5, ES6+ and ESNext
Not much to take notes on but just a deeper dive into the history of JavaScript/ ECMA and JavaScript Next which is considered the modern JavaScript. Goes on to mention how every year ECMA will release a new or update version of JavaScript and how the course will adapt to adding new changes into the course.